App Intents, or AI for the rest of us Apple Platforms developers.
I always found the framework intimidating, so this post is how I taught myself working with App Intents.
What is an Intent?
Let's go back to the basics, building the foundation first. What exactly is an intent?
An intent, in its simplest form, is a way of expressing a desired action or outcome. A user's wish or an action that can be understood by the OS and acted upon. For example, when you tell Siri, "Set an alarm for 7 AM," you are expressing an intent to create an alarm.
From Intent to App Intents
Intents are actions. Then, an app intents are the app's actions. In the Apple Platforms world, App Intents are your app's actions exposed to the system. Extending the actions of your app to iOS, Siri, and the Shortcuts app.
A quote that comes to my mind from a WWDC session I watched:
Anything your app does should be an App Intent.
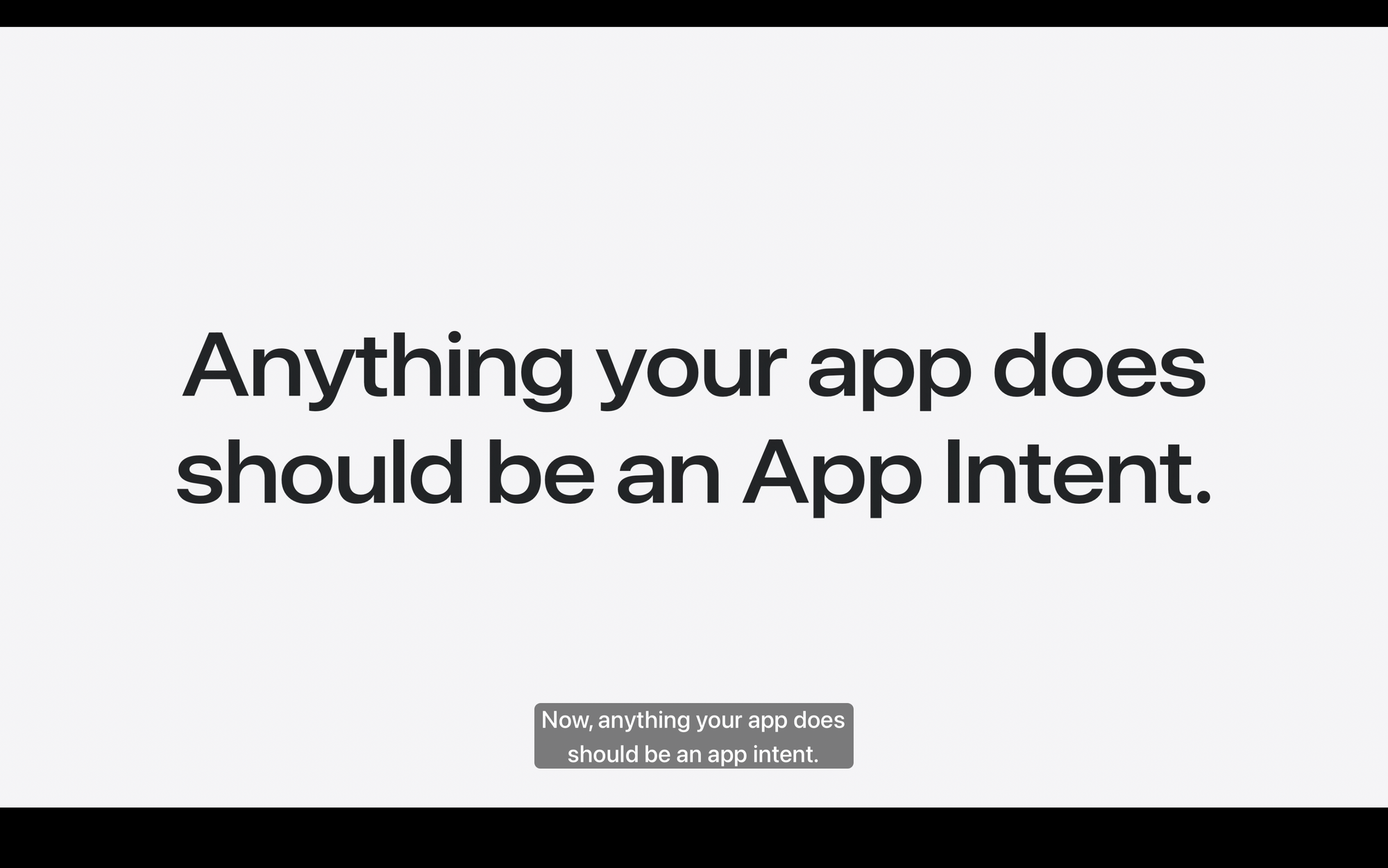
Now, we are not supposed to go about converting each method into an intent. But a general idea is to expose more of the app's functionality outside of the app itself. But why is this important?
It makes life easier for your users. They can do action with your app without even opening it. Imagine telling Siri to book a ride or add a task to your to-do list. Your app becomes part of a bigger picture, working at OS level with Siri, Shortcuts, and Spotlight.
App Intents can make your app more discoverable. Users might stumble upon new ways to use your app through Shortcuts or Siri suggestions. Can we think of it as free advertising? Indeed.
And with Widgets, and Apple Intelligence, we see that App Intent is future-proofing your app. As Apple keeps adding up new features, your app with solid App Intents are always ready; or you as a developer with the knowledge of how to work with them.
Starting From the Protocol: AppIntent
AppIntent
is a protocol provided by the AppIntents
framework.
@available(macOS 13.0, iOS 16.0, watchOS 9.0, tvOS 16.0, *)
public protocol AppIntent : PersistentlyIdentifiable, _SupportsAppDependencies, Sendable {
}
It is the foundation for creating intents that can be used with Siri and the Shortcuts app. By conforming to this protocol, we are telling the system that, "Hey, here is something my app can do!"
Creating Your First AppIntent
We will create an intent that simply opens the app. Yep, nothing fancy right now.
The journey to Apple Intellgence will be taken one step at a time. Here is what it looks like:
import AppIntents
struct OpenAppIntent: AppIntent {
static var title: LocalizedStringResource = "Open My App"
func perform() async throws -> some IntentResult {
.result()
}
}
Now, let's dissect this code and understand each part:
static var title: LocalizedStringResource = "Open My App"
The title
is a static property that provides a human-readable name for your intent. This is what users will see in Siri or the Shortcuts app. It is of type LocalizedStringResource
, which means it can be easily localized if your app supports multiple languages.
func perform() async throws -> some IntentResult
The perform()
method is called when your intent is executed. In the simple example, we are not doing anything except returning a result, but this is where you would put the code to actually open your app or perform any other action.
The IntentResult
is what your perform()
method returns. It indicates the outcome of your intent's execution. In this simple case, we are using .result()
, which means "the intent was performed successfully."
Moving Forward
I know. This was a simple example. But, combine it with Intent Driven Development for the actions in your app, and you are on the way to build something powerful.
Expose those actions outside of the app, and you have the power of Siri, Shortcuts, Widgets, Controls, and Action button!